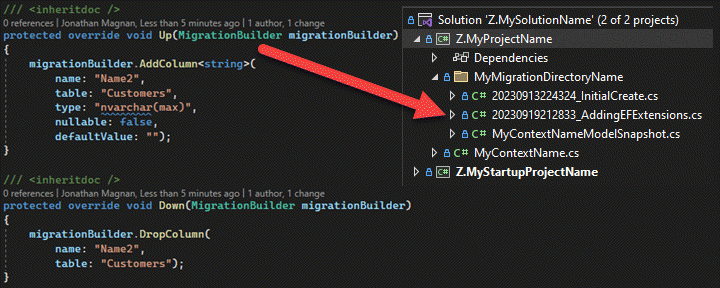
When using the Add-Migration command in EF Core, it generates three key files:
- [Timestamp]_[MigrationName].cs: The primary migration file with the
Up()
andDown()
methods. TheUp()
method has the code for when a migration is applied, and theDown()
method has the code for when a migration is reverted. - [Timestamp]_[MigrationName].Designer.cs: A snapshot of your model made using a fluent-api.
- [ContextClassName]ModelSnapshot.cs: The latest snapshot of your current model. The code is the same as the latest
[Timestamp]_[MigrationName].Designer.cs
produced. This file is made only during the initial migration and updated for every following migration.
The [Timestamp]
element is derived from the date and time when the migration is created, serving as a unique identifier for that specific migration instance.
This article will focus on the [Timestamp]_[MigrationName].cs file. You can view this Model Snapshot article to learn more about the snapshot generated by EF Core.
Changes Made:
- Changed "utilizing" to "using" for simpler vocabulary.
- Changed "concentred about" to "focus on" for clearer meaning.
- Made minor changes for clarity without rephrasing the overall content.
Main Migration File
The main migration file typically contains the two principal methods:
- Up Method: Contains changes to be made to the database when applying a migration.
- Down Method: Contains changes for when you need to undo or revert a migration.
Actions associated with this file:
- Added: By the Add Migration command.
- Removed: Via the Remove Migration command.
- Executed: Through the Update Database command.
Below is an example showcasing the contents of this file:
using Microsoft.EntityFrameworkCore.Migrations;
#nullable disable
namespace Z.MyProjectName.MyMigrationDirectoryName
{
/// <inheritdoc />
public partial class AddColumn : Migration
{
/// <inheritdoc />
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.AddColumn<string>(
name: "Name",
table: "Customers",
type: "nvarchar(255)",
nullable: false,
defaultValue: "");
}
/// <inheritdoc />
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropColumn(
name: "Name",
table: "Customers");
}
}
}
In the example above, a Name
property has been added to the Customer
entity:
- Up Method: The
Up
method contains the code to add a new column namedName
of typenvarchar(255)
to the 'Customers' table. - Down Method: The
Down
method contains the code to undo the actions of theUp
method, essentially removing theName
column from the 'Customers' table.
Up Method
The Up
method contains instructions to apply the desired changes, transitioning the database to a newer state.
For instance, consider a Name
property has been added to the Customer
entity. When using the Add-Migration command, a new migration file will be created with the Up
method that would encapsulate the code to introduce this new column to the database. Here is an example of what the Up
method could contain:
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.AddColumn<string>(
name: "Name",
table: "Customers",
type: "nvarchar(255)",
nullable: false,
defaultValue: "");
}
In this example, the AddColumn
method specifies the addition of a "Name" column to the "Customers" table. The new column is of string
type, cannot be null, and has a maximum length of 255 characters.
Down Method
The Down
method contains instructions to reverse the actions of the Up
method, reverting the database back to its previous state.
For instance, referring back to our previous example where the Name
property was added, to revert this change, we would need to remove the column from our database. Here is an example of what the Down
method could contain:
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropColumn(
name: "Name",
table: "Customers");
}
In this example, the DropColumn
method indicates that the "Name" column will be removed from the "Customers" table.
Available Methods
Inside the migration files, you can find multiple methods that are either part of:
- MigrationBuilder Methods
- Specific Builder Methods:
- OperationBuilder Methods
- AlterOperationBuilder Methods
- CreateTableBuilder Methods
- Provider Specific Methods:
- MySQL Methods (MySql.Data.EntityFrameworkCore)
- MySQL Methods (Pomelo.EntityFrameworkCore.MySql)
- PostgreSQL Methods
- SQLite Methods
- SQL Server Methods
MigrationBuilder Methods
This builder can be located in the MigrationBuilder.cs file on GitHub.
Add
- AddColumn
: Adds a column to a table. The T
represents the column's CLR type. - AddCheckConstraint: Adds a constraint to a table.
- AddForeignKey: Adds a foreign key to a table.
- AddPrimaryKey: Adds a primary key to a table.
- AddUniqueConstraint: Adds a unique constraint to a table.
Alter
- AlterColumn
: Alters a column of a table. The T
represents the column's CLR type. Returns an AlterOperationBuilder builder. - AlterDatabase: Alters a database. Returns an AlterOperationBuilder builder.
- AlterSequence: Alters a sequence of a table. Returns an AlterOperationBuilder builder.
- AlterTable: Alters a table of a database. Returns an AlterOperationBuilder builder.
Create
- CreateIndex: Creates an index for a table.
- CreateSequence: Creates a sequence for a table.
- CreateSequence
: Creates a sequence for a table. The T
represents the sequence's CLR type. - CreateCheckConstraint: Obsolete: Use
AddCheckConstraint
instead. Creates a check constraint for a table. - CreateTable
: Creates a table in a database. The TColumns
is usually an anonymous type that contains types for every column. Returns a CreateTableBuilder builder.
Drop
- DropColumn: Drops a column from a table.
- DropForeignKey: Drops a foreign key from a table.
- DropIndex: Drops an index from a table.
- DropPrimaryKey: Drops a primary key from a table.
- DropSchema: Drops a schema from a database.
- DropSequence: Drops a sequence from a table.
- DropCheckConstraint: Drops a check constraint from a table.
- DropTable: Drops a table from a database.
- DropUniqueConstraint: Drops a unique constraint from a table.
Rename
- RenameColumn: Renames a column in a table.
- RenameIndex: Renames an index in a table.
- RenameSequence: Renames a sequence in a table.
- RenameTable: Renames a table in a database.
CRUD
- InsertData: Inserts one or multiple data entries into a table.
- DeleteData: Deletes one or multiple data entries from a table.
- UpdateData: Updates one or multiple data entries in a table.
Misc
- EnsureSchema: Ensures a schema exists in a database. The schema will only be created if it doesn't already exist.
- RestartSequence: Restarts a sequence in a table to a specific value.
SQL
- Sql: Executes any SQL statement in the database. The SQL statement could be
insert
,update
,delete
, or any other SQL that is required for yourUp
orDown
method.
Specific Builder Methods
OperationBuilder Methods
This builder can be located in the OperationBuilder.cs file on GitHub.
This builder is returned by any other methods not returning an AlterOperationBuilder
or CreateTableBuilder
builder.
It contains the following methods:
- Annotation: Annotates the operation with the specified name/value pair.
AlterOperationBuilder Methods
This builder can be located in the AlterOperationBuilder.cs file on GitHub.
This builder is returned when invoking one of the following methods:
AlterColumn<T>
AlterDatabase
AlterSequence
AlterTable
It consists of the following methods:
- Annotation: Annotates the operation with the specified name/value pair.
- OldAnnotation: Annotates the operation with the name/value pair as it appeared before the alteration.
CreateTableBuilder
This builder can be located in the CreateTableBuilder.cs file on GitHub.
This builder is returned upon calling the following method:
CreateTable<TColumns>
It offers the following methods:
- Annotation: Annotates the operation with the specified name/value pair.
- ForeignKey: Adds a foreign key for the table.
- PrimaryKey: Adds a primary key for the table.
- CheckConstraint: Adds a check constraint to the table.
- UniqueConstraint: Adds a unique constraint for the table.
Provider Specific Methods
MySQL Methods (MySql.Data.EntityFrameworkCore)
Unfortunately, the code for MySql.Data.EntityFrameworkCore isn't open source. We couldn't find the source of MySql
extensions for migration when decompiling the DLL.
However, several sources mention two specific extensions for migrations. These were discovered in the MySQLMigrationsSqlGenerator.cs
file when decompiling and are also referenced in the MySQL Connector documentation.
IsMySql
: Returnstrue
if the active provider for the migration isMySQL
.CharSet
: Determines the charset for an entity property.Collation
: Determines the collation for an entity property.
MySQL Methods (Pomelo.EntityFrameworkCore.MySql)
You can find specific Pomelo.EntityFrameworkCore.MySql extensions for migration in the MySqlMigrationBuilderExtensions.cs file on GitHub. As of 2023, it has the following methods:
- IsMySql: Returns
true
if the active provider for the migration isMySQL
. - DropPrimaryKey: Removes a specific primary key for a table.
- DropUniqueConstraint: Removes a specific unique constraint for a table.
PostgreSQL Methods
You can find PostgreSQL
extensions for migration in the NpgsqlMigrationBuilderExtensions.cs file on GitHub. As of 2023, it has the following methods:
- IsNpgsql: Returns
true
if the active provider for the migration isPostgreSQL
. - EnsurePostgresExtension: Adds the
PostgreSQL
extension if it doesn't already exist. - CreatePostgresExtension: Obsolete; Use
EnsurePostgresExtension
instead. - DropPostgresExtension: Obsolete; Used to remove a PostgreSQL extension.
SQLite Methods
You can find SQLite
extensions for migration in the SqliteMigrationBuilderExtensions.cs file on GitHub. As of 2023, it has the following method:
- IsSqlite: Returns
true
if the active provider for the migration isSQLite
.
SQL Server Methods
You can find SQL Server
extensions for migration in the SqlServerMigrationBuilderExtensions.cs file on GitHub. As of 2023, it has the following methods:
- IsSqlServer: Returns
true
if the active provider for the migration isSQL Server
orAzure SQL databases
.